Attribute pickup process
To pick up user attributes from PingFederate, configure your application to authenticate itself, provide the reference ID, and store the user attributes from the response. This lets you get the user information needed to create a session in your application.
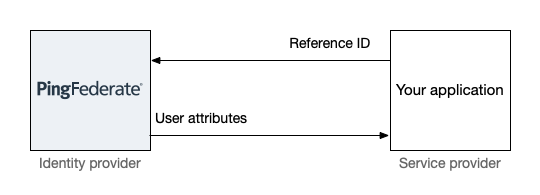
Requesting the user attributes
Configure your application to make an HTTP GET call to the Reference ID Adapter pickup endpoint:
https://pf_host:pf_port/ext/ref/pickup
In the call, provide the following:
-
The
REF
value. -
Authentication credentials, if you are using the HTTP Basic or HTTP header authentication. Learn more in Authentication methods.
-
The ID of the Reference ID Adapter instance.
Example code:
// Call back to PF to get the attributes associated with the reference String pickupLocation = "https://localhost:9031/ext/ref/pickup?REF=" + referenceValue; System.out.println(pickupLocation); URL pickUrl = new URL(pickupLocation); URLConnection urlConn = pickUrl.openConnection(); HttpsURLConnection httpsURLConn = (HttpsURLConnection)urlConn; httpsURLConn.setSSLSocketFactory(socketFactory); urlConn.setRequestProperty("ping.uname", "changeme"); urlConn.setRequestProperty("ping.pwd", "please change me before you go into production!"); urlConn.setRequestProperty("ping.instanceId", "spadapter");
Parsing the attributes from the response
The response from PingFederate includes user attributes from earlier in the authentication flow. They are encoded as a JSON object or as properties using the java.util.Properties
class.
Your adapter instance configuration determines which attributes are included in the response and how they are formatted. See the Send Request Parameters and Outgoing Attribute Format settings. |
Example response:
HTTP/1.1 200 OK { "authnCtx":"urn:oasis:names:tc:SAML:2.0:ac:classes:unspecified", "partnerEntityID":"company:saml20:idp", "subject":"jsmith", "instanceId":"sample_adapter", "sessionid":"sFMcTOaropYv5gYQZi1ZOpX7DZ8", "authnInst":"2013-03-28 20:42:10-0500" }
Configure your application to parse the response and store the user attributes.
Example code:
// Get the response and parse it into another JSON object which are the 'user attributes'. // This example uses UTF-8 if encoding is not found in request. String encoding = urlConn.getContentEncoding(); InputStream is = urlConn.getInputStream(); InputStreamReader streamReader = new InputStreamReader(is, encoding != null ? encoding : "UTF-8"); JSONParser parser = new JSONParser(); JSONObject spUserAttributes = (JSONObject)parser.parse(streamReader); System.out.println("User Attributes received = " + spUserAttributes.toString());